Practical Question: To-Do List Application
Create a simple to-do list application using HTML, CSS, and JavaScript. The application should allow users to:
- Add new to-do items.
- Mark items as completed.
- Remove items from the list.
- Persist the to-do list in the browser’s local storage so that the list remains after refreshing the page.
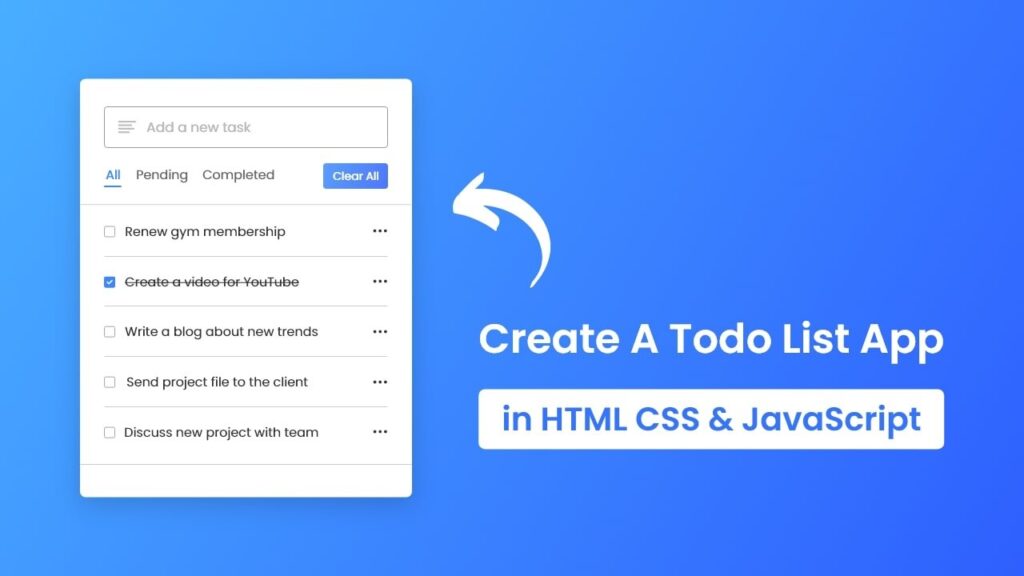
Solution
Step 1: Create the HTML structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>To-Do List</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>To-Do List</h1>
<input type="text" id="new-todo" placeholder="Add a new task">
<button id="add-btn">Add</button>
<ul id="todo-list"></ul>
</div>
<script src="script.js"></script>
</body>
</html>
- Head Section: Contains metadata, the title of the document, and a link to the CSS file for styling.
- Body Section: Includes a container div with an input field for new tasks, a button to add tasks, and an unordered list to display the tasks.
Step 2: Add CSS for styling
/* styles.css */
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
width: 300px;
}
h1 {
text-align: center;
}
#new-todo {
width: 80%;
padding: 10px;
margin-bottom: 10px;
}
#add-btn {
padding: 10px;
width: 20%;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: flex;
justify-content: space-between;
padding: 10px;
background-color: #f9f9f9;
margin-bottom: 5px;
border-radius: 3px;
}
.completed {
text-decoration: line-through;
color: gray;
}
- Body Styling: Centers the content on the page and sets a background color.
- Container Styling: Styles the main container with padding, background color, border radius, and shadow.
- Input and Button Styling: Styles the input field and button for adding tasks.
- List and List Item Styling: Styles the list and its items, including a class for completed tasks.
Step 3: Write JavaScript for functionality
// script.js
// Select DOM elements
const newTodoInput = document.getElementById('new-todo');
const addBtn = document.getElementById('add-btn');
const todoList = document.getElementById('todo-list');
// Load existing todos from localStorage
document.addEventListener('DOMContentLoaded', loadTodos);
// Add new todo
addBtn.addEventListener('click', addTodo);
// Add todo function
function addTodo() {
const todoText = newTodoInput.value.trim();
if (todoText !== '') {
const todoItem = createTodoItem(todoText);
todoList.appendChild(todoItem);
saveTodoToLocalStorage(todoText);
newTodoInput.value = '';
}
}
// Create todo item element
function createTodoItem(todoText) {
const li = document.createElement('li');
li.textContent = todoText;
const completeBtn = document.createElement('button');
completeBtn.textContent = 'Complete';
completeBtn.addEventListener('click', () => {
li.classList.toggle('completed');
});
const removeBtn = document.createElement('button');
removeBtn.textContent = 'Remove';
removeBtn.addEventListener('click', () => {
todoList.removeChild(li);
removeTodoFromLocalStorage(todoText);
});
li.appendChild(completeBtn);
li.appendChild(removeBtn);
return li;
}
// Save todo to localStorage
function saveTodoToLocalStorage(todoText) {
let todos = localStorage.getItem('todos') ? JSON.parse(localStorage.getItem('todos')) : [];
todos.push(todoText);
localStorage.setItem('todos', JSON.stringify(todos));
}
// Remove todo from localStorage
function removeTodoFromLocalStorage(todoText) {
let todos = localStorage.getItem('todos') ? JSON.parse(localStorage.getItem('todos')) : [];
todos = todos.filter(todo => todo !== todoText);
localStorage.setItem('todos', JSON.stringify(todos));
}
// Load todos from localStorage
function loadTodos() {
let todos = localStorage.getItem('todos') ? JSON.parse(localStorage.getItem('todos')) : [];
todos.forEach(todoText => {
const todoItem = createTodoItem(todoText);
todoList.appendChild(todoItem);
});
}
Explanation
Detailed Explanation
- Select DOM elements:
newTodoInput
,addBtn
, andtodoList
are selected usinggetElementById
to manipulate them later.
- Load existing todos from localStorage:
- When the DOM content is loaded,
loadTodos
function is called to populate the list with any previously saved tasks.
- When the DOM content is loaded,
- Add new todo:
addBtn.addEventListener('click', addTodo)
adds a click event listener to the “Add” button, callingaddTodo
function when clicked.
- Add todo function:
addTodo()
function gets the text from the input field, trims it, and checks if it’s not empty.- If the input is valid, it calls
createTodoItem(todoText)
to create a new list item and appends it to thetodoList
. - It then saves the new task to localStorage and clears the input field.
- Create todo item element:
createTodoItem(todoText)
creates a newli
element for the task.- It creates “Complete” and “Remove” buttons and adds click event listeners to toggle the completed class and remove the item, respectively.
- The buttons are appended to the
li
element, which is then returned.
- Save todo to localStorage:
saveTodoToLocalStorage(todoText)
retrieves the current list of todos from localStorage (or initializes an empty array if none exist).- It adds the new task to the array and saves it back to localStorage.
- Remove todo from localStorage:
removeTodoFromLocalStorage(todoText)
retrieves the current list of todos from localStorage.- It filters out the task to be removed and saves the updated array back to localStorage.
- Load todos from localStorage:
loadTodos()
retrieves the list of todos from localStorage and creates a list item for each saved task, appending it to thetodoList
.
Important Topic Of Javascript With Syntax Example and w2schools.com